アプリ開発をしているとほぼ必ず必要となるメールの送信機能。今回はこのメールの送信機能を追加してみる。
準備
まずはLaravelのプロジェクトを作成しよう。
composer create-project laravel/laravel MailTestLaravel
ルーティングの設定
route/web.php
Route::get('/', function () {
return view('index'):
});
ページの設定
今回はボタンを押したらメールが送信されるようにしたいのでLivewireを使用する。
composer require livewire/livewire
php artisan make:livewire MailTest
ボタンを作成する
<div>
<button wire:click='mail'>送信</button>
</div>
<?php
namespace App\Livewire;
use Illuminate\Support\Facades\Mail;
use Livewire\Component;
use App\Mail\TestMail;
class Test extends Component
{
public function mail(){
}
public function render()
{
return view('livewire.test');
}
}
とりあえずmailという名前のファンクションを作成しておくところまでやっておきます。
実際にメールを作成してみよう
まずはメールクラスを以下のコマンドで作成します。
php artisan make:mail SendTestMail
今回はSendTestMailという名前でクラスを作成してみました。実際に使用する場合はわかりやすい名前にすると良いと思います。
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Mail\Mailables\Content;
use Illuminate\Mail\Mailables\Envelope;
use Illuminate\Queue\SerializesModels;
use Illuminate\Mail\Mailables\Address;
class TestMail extends Mailable
{
use Queueable, SerializesModels;
public $mailData;
/**
* Create a new message instance.
*/
public function __construct($mailData)
{
$this->mailData = $mailData;
}
/**
* Get the message envelope.
*/
public function envelope(): Envelope
{
return new Envelope(
from: new Address('[email protected]', 'Test Account'),
subject: 'Test Mail',
);
}
/**
* Get the message content definition.
*/
public function content(): Content
{
return new Content(
view: 'mails.test',
);
}
/**
* Get the attachments for the message.
*
* @return array<int, \Illuminate\Mail\Mailables\Attachment>
*/
public function attachments(): array
{
return [];
}
}
今回は上記のようなプログラムにしてみました。それぞれ詳しく見てみようと思います。
public $mailData
public function __construct($mailData)
{
$this->mailData = $mailData;
}
この行ではコントローラーから与えられたデータをクラス内のmailDataという変数に入れているものでいわゆる初期化をしているところです。
public function envelope(): Envelope
{
return new Envelope(
from: new Address('[email protected]', 'Test Account'),
subject: 'Test Mail',
);
}
ここでは宛名を事前に用意するものです。他にもreplayToがあります。もしreplayToを使用する場合には以下のようにします。
public function envelope(): Envelope
{
return new Envelope(
from: new Address('[email protected]', 'Test Account'),
subject: 'Test Mail',
replyTo: [
new Address('[email protected]', 'Taylor Otwell'),
],
);
}
詳しくは下の公式ドキュメントを見てください。
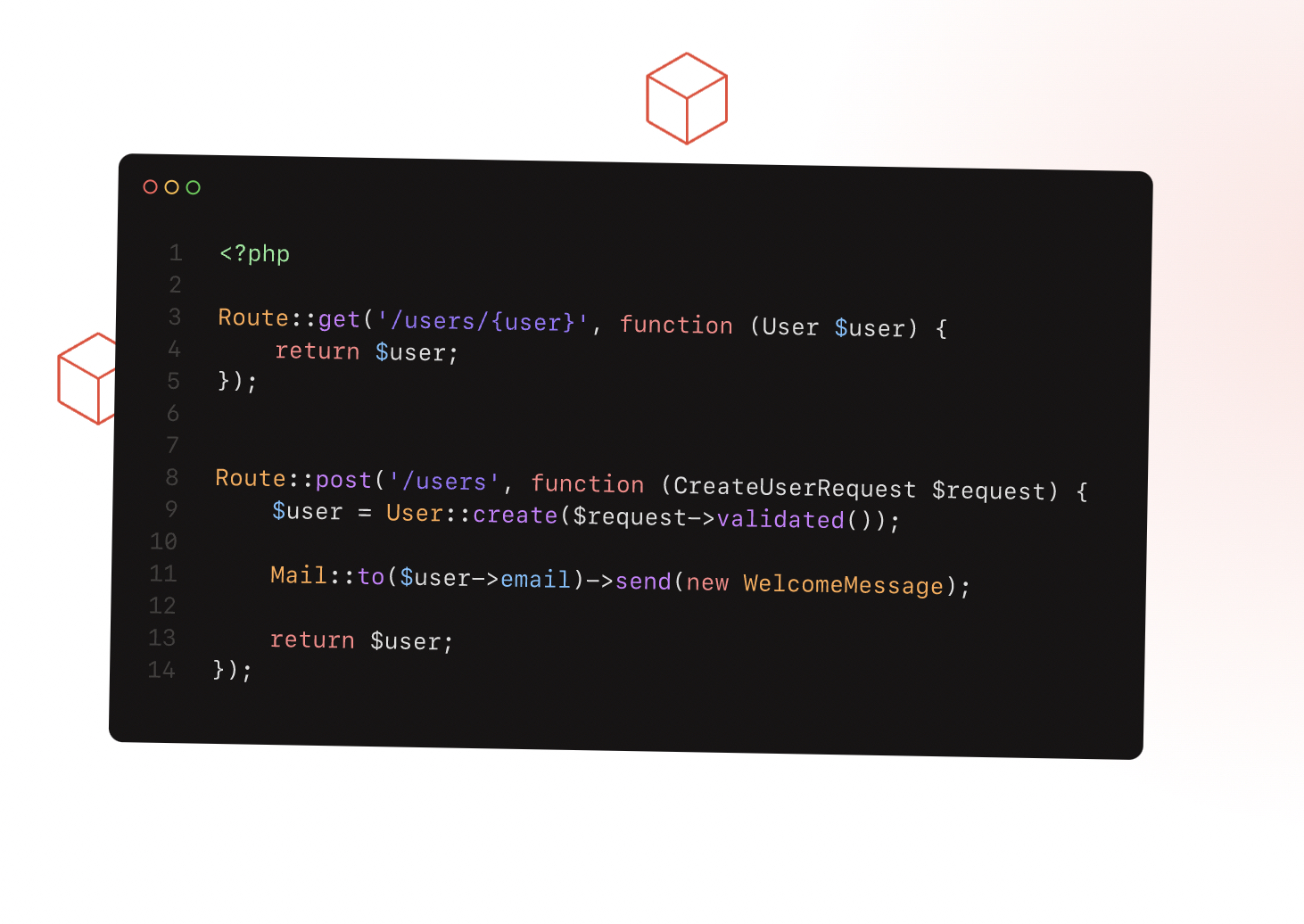
public function content(): Content
{
return new Content(
view: 'mails.test',
);
最後は実際にメールが入る本文です。上記のように指定をすれば、resources/views/mails/test.blade.phpの内容が送信されます。
もしデータをメール内のviewにわたすには以下のようにwithにデータを渡せばメールのviewで使えるようになります。
public function content(): Content
{
return new Content(
view: 'mails.test',
with: [
'data' => 'data',
],
);
}
<span>{{$data}}</span>
コントローラで実際に使用する
public function mail(){
Mail::mailer('default')->to('[email protected]')->send(new TestMail($mailData));
}
mailer:別のところにあるconfig/mail.phpにあるどのプロファイルを使用するのかを占めるところである。
to:メールの宛名を指定するところ
send:ここでメールクラスを指定する。ある意味このメールクラスはテンプレートみたいなもの。
ちなみに
config/mail.php
'mailers' => [
'default' => [
'transport' => 'smtp',
'host' => env('MAIL_HOST', 'smtp.mailgun.org'),
'port' => env('MAIL_PORT', 587),
'encryption' => env('MAIL_ENCRYPTION', 'tls'),
'username' => env('MAIL_USERNAME'),
'password' => env('MAIL_PASSWORD'),
'timeout' => null,
'auth_mode' => null,
]
],
.env
MAIL_MAILER="smtp"
MAIL_HOST="mail.ktr-server.com"
MAIL_PORT=587
MAIL_USERNAME="" //非公開
MAIL_PASSWORD="" //非公開
MAIL_ENCRYPTION="tls"
# MAIL_FROM_ADDRESS="[email protected]"
# MAIL_FROM_NAME="${APP_NAME}"
MAIL_MAILER="smtp"
MAIL_HOST="mail.ktr-server.com"
MAIL_PORT=587
MAIL_USERNAME="" //非公開
MAIL_PASSWORD="" //非公開
MAIL_ENCRYPTION="tls"
# MAIL_FROM_ADDRESS="[email protected]"
# MAIL_FROM_NAME="${APP_NAME}"
このようなテスト環境で行った。時前で作成したメールサーバーを利用しているので少し違いがあるのかもしれない。
コメント